What do you learn
- Understand how to make custom like button in WordPress without any plugin
- How to make like button without user log in
- How to fetch user IP and use it as user id in the site
- How to make a site to make anonymous like post & comments
Hello folks, Welcome to Mavnty. In this post you will know how to add custom like button or product Wishlist easily without any plugin. I will also provide detail code.
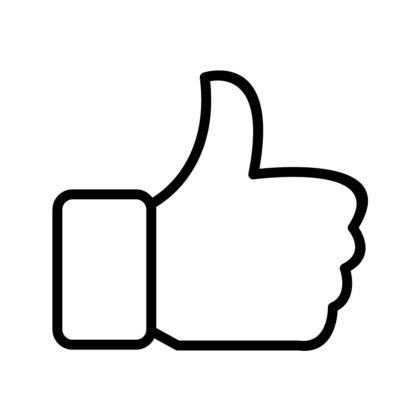
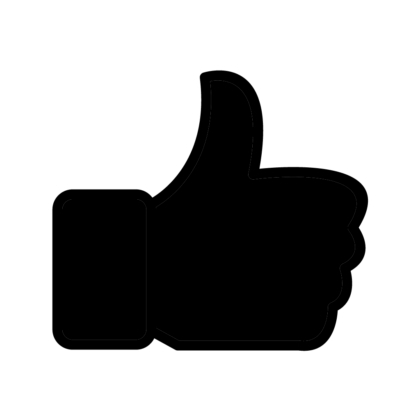
How WordPress Custom Like Button Works
Almost every site the like button or Wishlist button is used, For blog site you can use it for liking the blog post or liking the comments like that and for product based site user can like the product or make a Wishlist by this button. So for Functionality of this custom like button follow the steps.
I am doing it for two way, One, when a user is logged in and if a user is liking a post or give love react to a product it automatically store to their account that way they can save and check out their Wishlist in future.
The way is two like a post anonymously. So here we cannot get user id (Because the user is not logged in). In that case you can use cookie or IP address of the user to make user ID. I will show how to fetch user IP address and stored it as user id.
If you also check out detail tutorial of Custom Based WordPress Pagination Without Any Plugin, I provide detail code there.
WordPress Like Button Details
The code provided is based on three files custom.js for writing ajax code for liking and disliking, functions.php for ajax function. and here I use single.php for templating the like button.
Functions PHP code
Firstly enqueue custom.js file in functions.php and localize it into the enqueue function, the ajax url like below. Also need to localize a filled and empty like button image to it.
wp_localize_script( 'custom-js', 'ajax_posts', array(
'ajaxurl' => admin_url('admin-ajax.php'),
'noposts' => __('No older posts found', 'mywebsite'),
'emptyImageUrl' => get_template_directory_uri() . '/images/thumbsempty.png',
'filledImageUrl' => get_template_directory_uri() . '/images/thumbsfilled.png',
));
Put like button to WordPress template file, for that I am using Single.php for post like or dislike, you can use any of your custom templates.
<?php get_header(); ?>
<?php if(have_posts()): while(have_posts()): the_post(); ?>
<h2><?php the_title(); ?></h2>
<?php the_content(); ?>
<?php
$url = "https://api.ipify.org/?format=json";
$data = file_get_contents($url);
$result = json_decode($data, true);
$ip = $result['ip'];
$user_id = str_replace(".", "", $ip);
$liked = get_user_meta($user_id, '_liked', true);
$post_id = get_the_ID();
$watchlist = get_user_meta( $user_id, '_liked', true);
if( !empty( $watchlist ) )
{
$check_value = maybe_unserialize( $watchlist );
if(is_array($check_value) && !empty($check_value)){
if( in_array( $post_id, $check_value ) ){
echo "true".$post_id;
?>
<img class="thumbsempty" src="<?php echo get_template_directory_uri(); ?>/images/thumbsfilled.png" alt="">
<?php
}
else{
echo "false".$post_id;
?>
<img class="thumbsempty" src="<?php echo get_template_directory_uri(); ?>/images/thumbsempty.png" alt="">
<?php
}
}
else
{
?>
<img class="thumbsempty" src="<?php echo get_template_directory_uri(); ?>/images/thumbsempty.png" alt="">
<?php
}
}
else
{
?>
<img class="thumbsempty" src="<?php echo get_template_directory_uri(); ?>/images/thumbsempty.png" alt="">
<?php
}
?>
<p style="visibility: hidden;" class="postid"><?php echo get_the_ID(); ?></p>
<p class="count">(0)</p>
<?php endwhile; else: ?>
<h2><?php _e('Not Found')?></h2>
<p><?php _e('Sorry,no posts matched your criteria.'); ?></p>
<?php endif; ?>
<?php get_footer(); ?>
Here I use a API ipify JSON format to fetch user IP, if you want to fetch another way you can use this:
$ip = $_SERVER['REMOTE_ADDR'];
Ajax Code for WordPress Like Button
Now the time for the main ajax code, it is written on custom.js, just follow the code below
jQuery(document).ready(function ($) {
$(".thumbsempty").on("click", function () {
var $thumb = $(this);
$.getJSON("https://api.ipify.org/?format=json", function (e) {
var postID = $(".postid").text();
$.ajax({
url: ajax_posts.ajaxurl,
type: "post",
data: {
action: "my_ajax_action",
post_id: postID,
ip: e.ip,
},
success: function (response) {
if (response === "liked") {
$thumb.attr("src", ajax_posts.filledImageUrl);
} else {
$thumb.attr("src", ajax_posts.emptyImageUrl);
}
$("#result-container").html(response);
},
});
});
});
});
After that you have to put code in functions.php in respect to handle this ajax code
function my_ajax_action_handler() {
$post_id = $_POST['post_id'];
$user_id = $_POST['ip'];
$user_id = str_replace(".", "", $user_id);
$liked = get_user_meta($user_id, '_liked', true);
if (!empty($liked)) {
$check_value = unserialize($liked);
if (in_array($post_id, $check_value)) {
$arr_search = array_search($post_id, $check_value, true);
unset($check_value[$arr_search]);
update_user_meta($user_id, '_liked', serialize($check_value));
$action_result = 'unliked';
} else {
$check_value[] = $post_id;
update_user_meta($user_id, '_liked', serialize($check_value));
$action_result = 'liked';
}
} else {
$post_arr[] = $post_id;
update_user_meta($user_id, '_liked', serialize($post_arr));
$action_result = 'liked';
}
echo $action_result;
wp_die();
}
add_action('wp_ajax_my_ajax_action', 'my_ajax_action_handler');
add_action('wp_ajax_nopriv_my_ajax_action', 'my_ajax_action_handler');
The above code will fetch user IP from API or you can use this $ip = $_SERVER[‘REMOTE_ADDR’];
If you want to work with logged in user then you can use the below code both template and functions.php
<?php
$current_user_id = get_current_user_id();
?>
To check if the user logged in or not you can follow basic condition like
<?php
if (is_user_logged_in()) {
// User is logged in
echo "User is logged in.";
} else {
// User is not logged in
echo "User is not logged in.";
}
?>
By this way you can also run the above like and Wishlist functionalities for your WordPress site. I use user meta field a custom meta called _liked to store user like in respect of post id. I make the code in a serialize way to that every post like and dislike connected to every user.
Conclusion
Hope this articles helps you. Make sure you maintain GDPR rules and regulation to capture user details when it is annonymous user. If you need this functionalities with a plugin, I will definitely provide a detailed article on this in future. Stay tuned.